每日一题:用栈实现队列(LeetCode 232)
题目
请你仅使用两个栈实现先入先出队列。
队列应当支持一般队列支持的所有操作(push、pop、peek、empty):
实现 MyQueue 类:
- void push(int x)将元素 x 推到队列的末尾
- int pop() 从队列的开头移除并返回元素
- int peek() 返回队列开头的元素
- boolean empty()如果队列为空,返回 true ;否则,返回 false
解析
入队操作
如果是栈的插入操作,那我们可以把元素都先插入到 stackIn 中,也就实现了队列的 入队操作 。
出队操作
- 当 stackOut 中不为空时,直接操作,此时在 stackOut 中的栈顶元素是最先进入队列的元素,返回该元素即可;
- 如果 stackOut 为空且 stackIn 不为空,首先需要把 stackIn 中的元素逐个弹出并压入到 stackOut 中,然后返回 stackOut 的栈顶元素即可。
解答
class MyQueue {
public:
stack<int> stkIn;
stack<int> stkOut;
MyQueue() {
}
void push(int x) {
stkIn.push(x);
}
int pop() {
if(stkOut.empty())
{
while(!stkIn.empty())
{
stkOut.push(stkIn.top());
stkIn.pop();
}
}
int res = stkOut.top();
stkOut.pop();
return res;
}
int peek() {
if(stkOut.empty())
{
while(!stkIn.empty())
{
stkOut.push(stkIn.top());
stkIn.pop();
}
}
return stkOut.top();
}
bool empty() {
return stkIn.empty() && stkOut.empty();
}
};
/**
* Your MyQueue object will be instantiated and called as such:
* MyQueue* obj = new MyQueue();
* obj->push(x);
* int param_2 = obj->pop();
* int param_3 = obj->peek();
* bool param_4 = obj->empty();
*/
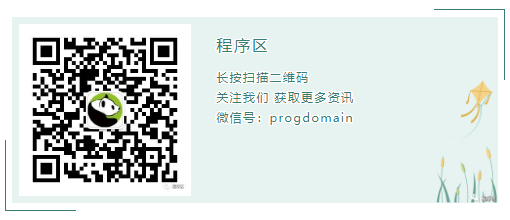
有任何问题,请联系邮箱:support@progdomain.com
THE END
0
二维码
打赏
海报
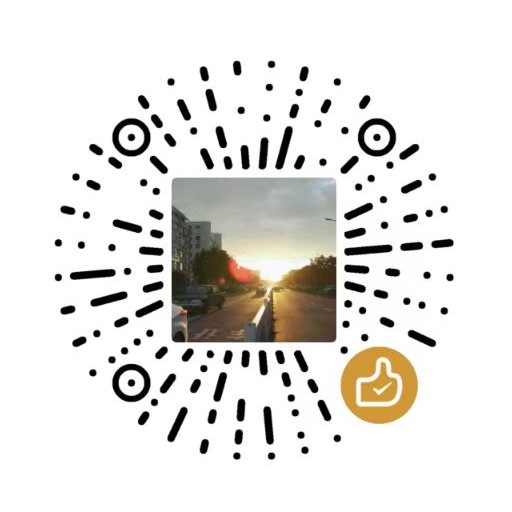
每日一题:用栈实现队列(LeetCode 232)
题目
请你仅使用两个栈实现先入先出队列。
队列应当支持一般队列支持的所有操作(push、pop、peek、empty):
实现 MyQueue 类:
void push(int x)将元素 x ……
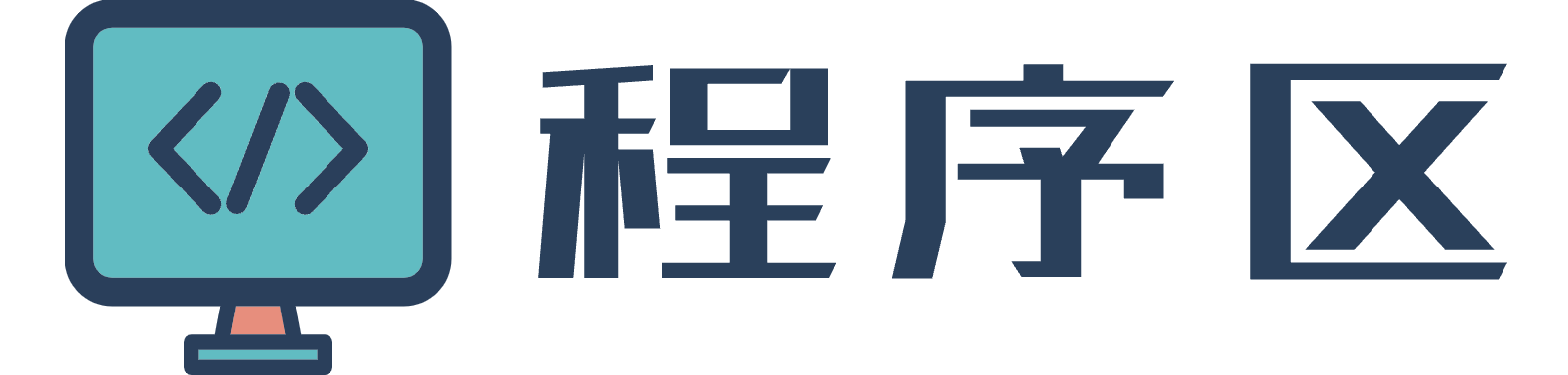
共有 0 条评论